
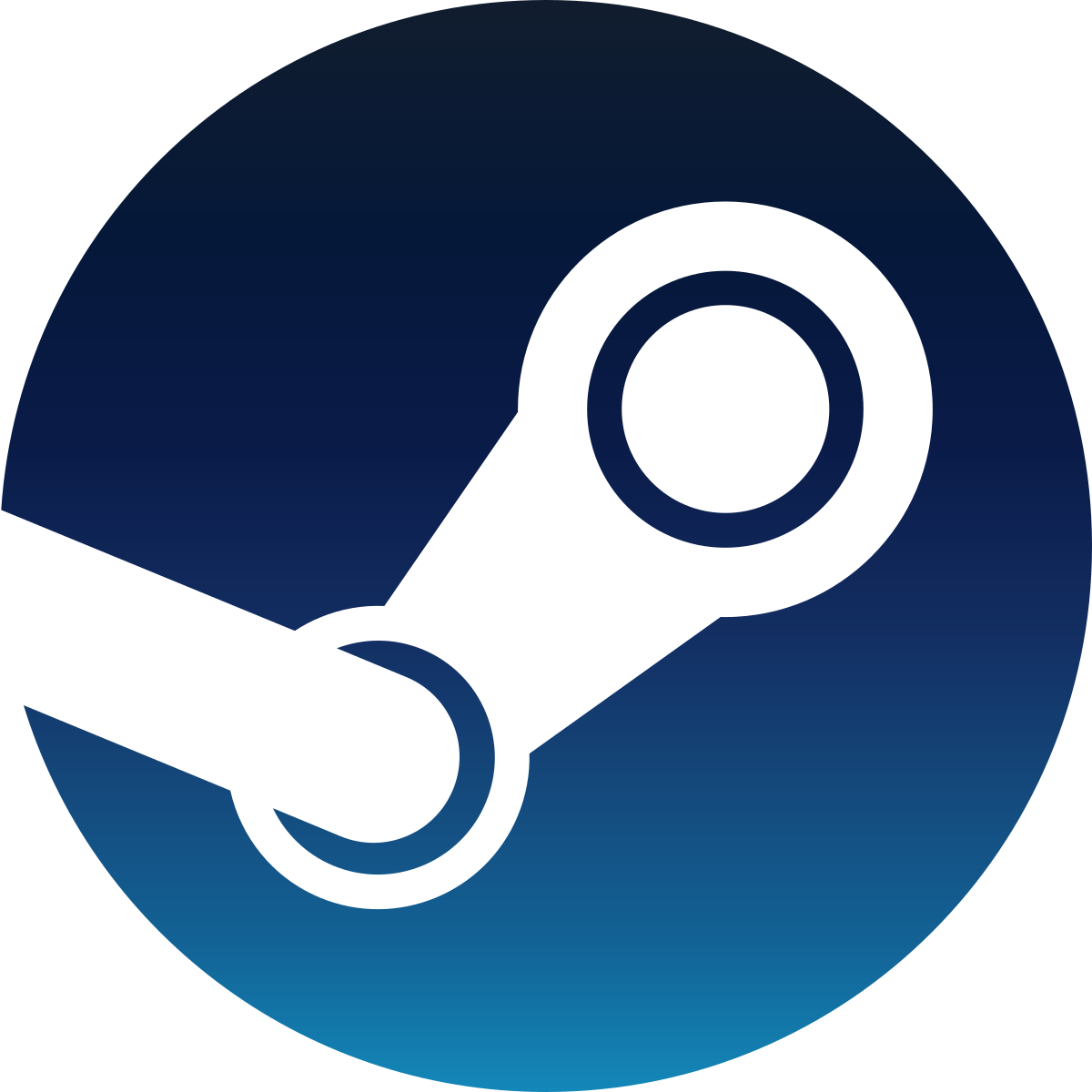
I have mine set up with a bunch of categories that are sorted with a prepended 3-digit number. Allows me to have different sections of category without it getting mixed up. ex:
010 S
011 A+
012 A
013 A-
014 B+
etc...
350 plz play soon
355 wont play
...
800 dont remember buying this
Darn that sucks to hear. I was somewhat active several months ago. Unfortunately the server never hit “critical mass”. My interest in any given game also waxes & wanes significantly so I know I kinda abruptly stopped playing.